What is Selenium?
Selenium is a popular open-source testing tool used for web application testing. It enables testers to write automated tests in various programming languages to test the functionality of web applications. Selenium tests can be run on many different browsers and operating systems.
Selenium Testing Tool is an excellent tool for automating web application testing. It can be used to test the functionality of web applications on various browsers and operating systems. Selenium Software Testing is a great way to automate your web application testing.
History of Selenium
Selenium’s journey began in 2004 when Jason Huggins, an engineer at ThoughtWorks, developed a JavaScript library called JavaScriptTestRunner to automate interactions with web applications. Recognizing the potential for a broader application, he open-sourced the tool, which was later renamed Selenium. The name was a light-hearted jab at a competitor’s product, Mercury Interactive, as selenium supplements are known to counteract mercury poisoning.
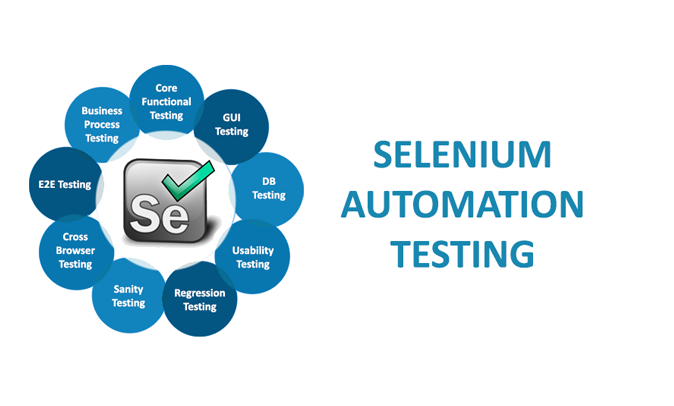
Key milestones in Selenium’s history include:
Selenium Remote Control (RC): Introduced to bypass the same-origin policy in browsers, allowing testers to write automated tests in various programming languages.
Selenium WebDriver: Launched to address limitations of Selenium RC by interacting directly with browser applications, providing a more robust and concise programming interface.
Selenium Grid: Developed to run concurrently across multiple machines and browsers, significantly reducing test execution time.
Selenium 2 and 3: Merged WebDriver and RC functionalities, enhancing stability and introducing support for newer web technologies.
Selenium 4: Released with W3C WebDriver standardization, offering new features like relative locators, an improved Selenium IDE, and better integration with modern browsers.
Who Uses Selenium?
Selenium is a go-to tool for many organizations due to its flexibility and support for multiple programming languages and browsers. Its users include:
Tech Giants: Companies like Google, Netflix, and HubSpot leverage Selenium for automated testing of their complex web applications.
Financial Institutions: Banks and financial services utilize Selenium to ensure the reliability and security of their online platforms.
E-commerce Platforms: Online retailers use Selenium to test user interfaces and shopping cart functionalities across browsers and devices.
QA and Testing Firms: Specialized firms employ Selenium to provide automated testing services to clients across various industries.
Educational Institutions: Universities and coding bootcamps incorporate Selenium into their curriculum to teach students about automated web testing.
Components of Selenium
Selenium is a collection that consists of three major components:
- Selenium IDE: Selenium IDE is a Firefox add-on that enables testers to record and playback the recorded automated tests. Selenium IDE is easy to use and lets you create and run automated tests quickly. Selenium IDE Chrome also includes a built-in debugger that enables you to troubleshoot your tests. To use Selenium IDE, you first need to install the Selenium add-on for Firefox or Chrome. You can then open Selenium IDE by clicking on the “Selenium” icon in the Firefox or Chrome toolbar.
Once Selenium IDE is open, you can start recording your tests by clicking on the “Record” button. Selenium will then begin recording all of your actions as you perform them in the browser. To stop the test recording, click on the “Stop” button. You can then playback your tests by clicking on the “Play” button. Selenium will then replay all of the actions that you recorded.
- Selenium WebDriver: Selenium WebDriver is an open-source tool used for automating web browser interaction from a user perspective. With it, you can write tests that simulate user interactions with a web application. It is available for many different programming languages, such as Java, C#, Python, and Perl.
WebDriver provides a powerful and flexible test automation framework that enables you to create automated tests for your web applications easily. It also includes several over-the-top features, such as automatically discovering elements on a web page and capturing screenshots of your tests.
- Selenium Grid: Selenium Grid distributes your tests across multiple machines or virtual machines (VMs). Selenium Grid enables you to test parallelly on various devices or VMs, allowing you to scale your test automation quickly. Selenium Grid is a crucial part of the overall Selenium testing suite and will enable you to execute your automated tests much faster.
The Selenium Server is a part of Selenium Grid that can be installed locally on your device or hosted on a separate system/server.
How does Selenium work?
Selenium WebDriver works by emulating the actions of a user. When you write a Selenium test, you specify the actions you want the user to take, and Selenium will automatically execute those actions on the browser.
For instance, if you need to test a login functionality, you would write a Selenium test to enter the username and password into the appropriate fields and click on the login button. Selenium would automatically execute those actions on the browser and report any errors.
Importance of testing in Selenium
Selenium has become a cornerstone in web application testing due to its flexibility, ease of use, and robust capabilities. Here are some key reasons why testing in Selenium is important:
Cross-Browser Compatibility: Selenium supports multiple browsers, including Chrome, Firefox, Safari, and Internet Explorer. This ensures your web application functions seamlessly across different browsers, providing a consistent user experience.
Supports Multiple Programming Languages: Selenium supports various programming languages such as Java, C#, Python, and Ruby. This allows testers to write test scripts in a language they are comfortable with, improving the efficiency and effectiveness of the testing process.
Integration with Other Tools: Selenium can easily integrate with other testing frameworks and tools like TestNG, JUnit, and Maven. This enhances the testing capabilities and allows continuous integration and delivery (CI/CD).
Open Source and Community Support: Selenium is an open-source tool that is free to use and has a large and active community. This community contributes to continuous improvement, provides support, and shares resources, making resolving issues easier and staying updated with the latest advancements.
Reusable Test Scripts: Selenium allows the creation of reusable test scripts for regression testing. This saves time and effort and ensures that new changes do not affect functionality.
Testing on Real Devices and Browsers: Selenium can be used with cloud-based platforms to test on real devices and browsers, providing accurate results and better coverage.
Why should every tester learn about Selenium WebDriver?
Selenium WebDriver is a widely used tool in software testing due to its versatility, ease of use, and support for various programming languages. It allows testers to automate browser interactions in real-world scenarios, making it ideal for cross-browser testing. Learning Selenium WebDriver provides testers with the ability to:
Automate Complex Web Applications: WebDriver supports interaction with dynamic web elements, enabling automated testing for complex, AJAX-heavy, and responsive web applications.
Support for Multiple Browsers: WebDriver supports major browsers like Chrome, Firefox, Safari, and Edge, making it a go-to tool for cross-browser testing, ensuring the application works uniformly across platforms.
Open-source and Community Support: Selenium WebDriver is open-source, and its vast community offers resources, libraries, and updates, which makes it easier to learn and troubleshoot.
Integration with CI/CD Pipelines: WebDriver integrates easily with Continuous Integration tools like Jenkins, making it crucial for maintaining robust, automated test suites in Agile and DevOps environments.
Career Advancement: Proficiency in Selenium WebDriver is a highly sought-after skill for manual and automation testers in the job market. Its prominence in testing frameworks makes it an essential tool for every tester.
The architecture of Selenium WebDriver
The architecture of Selenium WebDriver is built to automate web browsers across different platforms and programming languages. It follows a client-server model that interacts with browsers through browser-specific drivers. The key components of Selenium WebDriver’s architecture are:
Selenium Client Libraries: Selenium offers libraries for multiple languages like Java, Python, C#, and Ruby. These libraries enable testers to write automation scripts that can be executed across different browsers.
JSON Wire Protocol: Selenium WebDriver communicates with the browser using the JSON Wire Protocol over HTTP. The JSON Wire Protocol defines a standard way for WebDriver to interact with the browser, sending instructions from the client to the server.
Browser Drivers: Each browser has its own WebDriver that translates the commands from the client into actions that can be performed on the browser. For example, ChromeDriver is used for Chrome, while GeckoDriver is used for Firefox. These drivers launch the browser and execute the commands sent by WebDriver.
Selenium WebDriver: At the core of the architecture, Selenium WebDriver handles the communication between the client libraries and the browser drivers. It translates the code written by testers into a language that browsers can understand, allowing testers to automate actions like clicking, filling forms, and navigating between web pages.
Browsers: The browsers execute the commands they receive from WebDriver. The browser driver listens to commands from WebDriver and then performs the corresponding browser actions.
This architecture ensures Selenium WebDriver can seamlessly interact with browsers, regardless of the language or platform, making it highly adaptable for web testing.
Selenium automation testing tools
Selenium is a suite of tools that cater to different testing needs. Here are the main components of Selenium and how they contribute to automation testing:
Selenium WebDriver: WebDriver allows for interaction with web browsers. It drives the browser in a way that simulates a real user, making it ideal for automated testing of web applications. WebDriver supports various programming languages and can handle dynamic web elements efficiently.
Selenium IDE: Integrated Development Environment (IDE) is a simple tool for recording and playing back test scripts. It is useful for creating quick prototypes and test scripts without advanced programming knowledge. Selenium IDE is primarily used to create exploratory tests and small-scale automation tasks.
Selenium Grid: Selenium Grid allows for parallel execution of test scripts on multiple browsers. This distributed testing framework speeds up the testing process and ensures comprehensive test coverage across different environments. Selenium Grid is particularly beneficial for large projects requiring extensive testing.
Selenese: Selenese is the set of commands used in Selenium IDE to perform various operations, such as clicking a button, entering text, and verifying page elements. These commands form the basis of the test scripts and can be exported to different programming languages when moving from Selenium IDE to WebDriver.
Browser-Specific Drivers: Selenium WebDriver interacts with different web browsers using browser-specific drivers like ChromeDriver, GeckoDriver (for Firefox), and EdgeDriver. These drivers translate the WebDriver commands into actions performed by the browser, ensuring compatibility and accurate execution of test scripts.
How to get started with Selenium automation tool
With Selenium automation tool, you can write automated tests in various programming languages. This enables you to test the functionality of your web application on different browsers and operating systems. Selenium Testing is a great way to automate your web application testing.
To use the Selenium Testing Tool, you must install the Selenium WebDriver. It is a browser automation tool that enables you to run Selenium tests on different browsers and operating systems. Once you have successfully installed it, you can start writing your Selenium tests.
Installing Selenium is easy. You can install Selenium by following these steps:
Go to the Selenium website: https://www.selenium.dev/
Click on the “Download” button from the menu.
Select the “Selenium IDE” option and choose the browser from all the listed browsers.
Click on the “Download” button.
Run the “SeleniumIDE_4.0.0.exe” file or the latest build.
Follow the prompts to install Selenium IDE.
The Selenium will be installed, and you can start writing your Selenium tests.
When writing Selenium tests, you should always keep in mind the following:
The goal of your Selenium test is to find bugs in your web application.
Your Selenium tests should be concise.
You should only use the Selenium WebDriver When you are sure about how to use the tools and scripts.
Once you have written your Selenium tests, you can run them on different browsers and operating systems. To do this, you will need to use a Selenium Grid. A Selenium Grid is a server that enables you to run multiple Selenium tests simultaneously on different browsers and operating systems.
The Selenium benefits include:
Efficient and accurate web application testing.
The ability to test your web application on multiple browsers and operating systems.
The ability to run more than one test at the same time.
With a Selenium Grid, you can significantly reduce your time to test your web application. And by using a Selenium Grid, you can ensure that your web application is fully functional before releasing it to users. So, if you want to improve your web application testing, consider using a Selenium Grid. It’s one of the best ways to automate your web application testing!
Selenium in an agile environment
Selenium is an all-in-one tool that can help you streamline your agile testing process. By following the tips below, you can ensure that your automated tests are practical and efficient and that they play a valuable role in your agile development cycle:
Automated tests should be run frequently as part of the continuous integration process.
Tests should be written to allow them to be run quickly and easily.
Tests should be designed to test a specific functionality or behavior and should not be too complex.
New features and changes should be accompanied by automated tests to ensure that the application’s functionality remains intact.
Automated tests should supplement manual testing rather than replace it altogether.
In agile development, Selenium Testing is typically used in the following ways:
As part of the regression testing process, to ensure that existing features continue to work as expected after new code has been added.
To verify that new features are working as expected before they are released to production.
To help identify and troubleshoot bugs in web applications at both business and development levels before releasing the app.
Different types of Selenium tests
Selenium Testing is a popular open-source testing tool used for web application testing. It enables testers to write automated tests in various programming languages to test the functionality of web applications. Selenium tests can be run on many different browsers and operating systems.
There are different types of Selenium Tests that you can write. The most common types of Selenium Tests are:
Unit Tests
Functional Tests
Integration Tests
Regression Tests
Unit tests
Unit Tests are the simplest type of Selenium tests. A Unit Test verifies that a single unit of code behaves as expected.
When writing a Unit Test, you should first create a test case. A test case is a set of instructions that tests a particular feature or function of your code. To create a test case, you will need to:
Define the expected outcome of the test.
Verify that the code under test behaves as expected.
Once your test case is written, you can run it using the WebDriver. Open your browser and go to the page where your test is located. Then, enter your test case into the browser’s address bar and press “Enter.” Your Unit Test should automatically run, and you will be able to see if the code under test behaved as expected.
If you seem to be having any issue with a specific unit of code or need help troubleshooting a bug in your code, many online resources can help. Some useful resources include:
Tutorials and guides on Selenium Testing and Selenium for mobile testing from websites like Selenium.dev
Online communities and forums dedicated to Selenium Testing
Commercial support from companies like HeadSpin
Selenium Testing
In this example, we first opened the “selenium_testing.html” file in our web browser using the Selenium WebDriver. We then used the “webDriverWait” function to wait for a particular element, the “navbar_link” element, to be visible on the page before continuing with our test. After waiting for 30 seconds (as defined in the “implicitlyWait” timeouts), we verified that this condition occurred and executed our test code as expected.
Also check: A Comparative Guide to Automation and Manual Testing
Integration Tests
Integration Tests are used to test the integration between different parts of your web application. When writing an Integration Test, you should always keep in mind the following:
The goal of Integration Testing in Selenium is to find bugs in your web application that arise from integrating multiple components.
Your Integration Test should be short, and it should verify that all individual components work properly when integrated.
Example of writing integration test in Selenium
One useful feature of the WebDriver is its ability to wait for certain conditions before executing a test. This can be used, for example, to wait for a page to load or an element to show on the page before continuing with the test. You can use the “webDriverWait” function along with one of several different timeouts.
For example, you could use the “implicitlyWait” timeout, which will automatically wait for an indefinite time until a condition occurs before continuing with your test. Alternatively, you could use one of the other timeouts, such as “until,” which will only wait a specified amount of time before continuing your test. Using these features can help you improve the accuracy and efficiency of your Integration Tests by ensuring that they are executed properly every time.
To write an Integration Test in Selenium, you will first need to create a new file called “integration_test.html” in your test directory. This file will contain your test code, which will be executed in your web browser using the WebDriver.
Read: How digital automation is improving cost-efficiency for Telcos
Once you have created the “integration_test.html” file, you can begin writing your Integration Test. You will first need to include the WebDriver library in your HTML file to do this. You can do this by adding the line of code below to your script:
Next, you will need to write your test code. In this example, we will be testing the “login” functionality of a web application. First, we need to define the expected outcome of our test and then write the code that will verify that this outcome occurs. In this case, we expect the “login” button to be displayed on the page after entering our username and password.
Finally, we will need to use the “webDriverWait” function to wait for the “login” button to be visible on the page before continuing with our test. After waiting for 30 seconds (as defined in the “implicitlyWait” timeouts), we verified that this condition occurred and executed our test code as expected.
End-to-end tests
End-to-End Tests are used to test an entire web application from start to finish. When writing an end-to-end test, you should always keep in mind the following:
The goal of your End-to-End Test is to find bugs in your web application that arise as a result of the correlation between multiple components.
Your End-to-End Test should be concise, and it should verify that all individual components work properly when integrated.
Once your test cases are written, you can run them using the WebDriver. Open your browser and go to the page where your tests are located. Then, enter your test case into the browser’s address bar and press “Enter.” Your End-to-End Test should automatically run, and you will be able to see if the code under test behaved as expected.
End-to-end Selenium test example
To write an End-to-End Test in Selenium, you must create a new file called “end_to_end_test.html” in your test directory. This file will contain your test code, which will be executed in your web browser using the WebDriver.
Once you have created the “end_to_end_test.html” file, you can begin writing your End-to-End Test. You will first need to include the WebDriver library in your HTML file to do this. You can do it by adding the given line of code:
Next, you will need to write your test code using the WebDriver API functions such as “click,” “type,” and “waitForPageToLoad.” In this example, we are testing a simple login page that requires users to enter their username and password before accessing the application. We are then verifying that the expected outcome of our test occurred by checking if the login button is visible on the page after we have entered our credentials in the login form field.
Also read: Aligning Your Testing Approach with Your Product’s Maturity
Finally, we will use some of Selenium’s built-in timeouts to ensure that our End-to-End Test runs smoothly. For example, we will use the “implicitlyWait” timeout to wait for 30 seconds before continuing with our test code to allow any slow or loading pages to load completely. We will also use the “webDriverWait” function to wait for a specified period before executing our test code to ensure that all application components are fully loaded and working properly.
Tips for running successful Selenium Tests
Certain tips can help you write better selenium scripts and automate your application tests more efficiently. Some of these tips are:
Always start by writing a simple test case that verifies the functionality of a single component.
Keep your tests concise, and verify that all individual components work properly when integrated.
Use the Selenium WebDriver API functions such as “click”, “type”, and “waitForPageToLoad”.
Make sure to include timeouts in your test code to ensure that your tests run smoothly.
Use the Selenium Grid for parallel testing to speed up your tests’ execution.
Always run your tests on a variety of different browsers and operating systems.
Keep your test code well organized and maintainable to be easily reused and modified in the future.
Limitations of Selenium WebDriver
Although Selenium is one of the best tools for automating your tests on multiple devices, it still has limitations. Some of them are mentioned below:
WebDriver cannot interact with flash or Java applets.
WebDriver is not capable of handling complex animations.
WebDriver cannot recognize text inside images.
WebDriver has some difficulty dealing with dynamically generated pages.
WebDriver can be difficult to use when testing web applications that use Ajax or ReactJS.
Troubleshooting common issues with Selenium tests
Troubleshooting issues with Selenium Tests can be challenging, but a few techniques can help you identify and resolve these issues efficiently and quickly.
One of the best ways to troubleshoot Selenium Tests is using a Selenium Grid, which allows you to run your tests parallel on multiple browsers and operating systems. This can help you identify performance or compatibility problems that may not be visible when running your tests on a single browser or device.
In addition, it is vital to make use of all of the features available with the WebDriver, such as screenshots and video recording tools. These can be extremely useful for debugging purposes, as they allow you to easily capture detailed information about any errors or bugs that occur during your tests.
Finally, it is also essential to test for security vulnerabilities using the Selenium Security Testing Framework. This can help you locate and fix any potential security issues in your web applications.
Check out: A comprehensive guide to mobile app security testing
By following the tips mentioned, you can ensure that your Selenium Tests are running smoothly and efficiently.
Selenium WebDriver 4.0
The WebDriver 4.0 was released in October 2021 and included several new features and improvements. Some of the key features include:
Support for running Selenium tests on mobile devices, including Android and iOS. This allows testers to automate their mobile app testing using the same Selenium framework to test web applications.
The ability to run several tests parallelly on multiple browsers and operating systems using the improved version of Selenium Grid can significantly improve performance and efficiency.
New security features have been added to help you identify potential vulnerabilities in your web applications, such as SSL verification and certificate pinning.
Improved debugging tools make it easier to troubleshoot issues with your Selenium Tests and quickly resolve any bugs or errors during execution.
W3C Standardization: The WebDriver 4.0 has been standardized by the W3C, making it easier to use with other tools and frameworks.
Relative Locators: This new feature allows you to locate elements using relative coordinates, which can be especially useful when testing dynamic web applications.
Overall, the WebDriver 4.0 is a powerful tool that offers many new features and improvements that make it easier to automate your web application testing.
Additional resources on Selenium testing
There are many resources available on Selenium Testing. Some of these resources include:
The official documentation for the Selenium project: http://docs.seleniumhq.org/
The Selenium Blog: https://www.selenium.dev/blog/
The Selenium Wiki: https://github.com/SeleniumHQ/selenium/wiki
Online tutorials and courses on Selenium Automation Testing: https://www.udemy.com/topic/selenium-webdriver/free/
How to write an automated test script using Selenium: https://www.headspin.io/blog/how-to-write-an-automated-test-script-using-selenium
Selenium Automation Tips You Must Know: https://www.headspin.io/blog/selenium-automation-tips-you-must-know
Using XPath in Selenium: https://www.headspin.io/blog/using-xpath-in-selenium-effectively
Pros and Cons of Selenium: https://www.headspin.io/blog/pros-and-cons-of-selenium-in-automation-testing
Using WebDriverWait in Selenium: https://www.headspin.io/blog/using-webdriver-waits-in-selenium
Whether you are new to Selenium Mobile Testing or have been using this tool for some time, there’s a load of information available online to help you learn more about this powerful testing tool and improve your web application testing. So, make sure you take advantage of these resources!
Future of Selenium Testing
The future of Selenium testing is poised to evolve alongside advancements in web technologies and testing methodologies:
Integration with AI and Machine Learning: Selenium is expected to incorporate AI for smarter test automation, including self-healing tests that adapt to changes in the UI.
Enhanced Cloud Testing: Greater integration with cloud-based platforms will facilitate scalable and distributed testing environments, enabling testers to run extensive test suites more efficiently.
Support for New Web Frameworks: As web development evolves, Selenium will continue to update its support for emerging frameworks and technologies, such as Progressive Web Apps (PWAs) and WebAssembly.
Improved Developer Experience: Ongoing enhancements to the Selenium IDE and better documentation aim to make Selenium more accessible to newcomers while streamlining workflows for experienced testers.
Standardization and Compliance: With the adoption of W3C WebDriver standards, Selenium will offer more consistent behavior across different browsers, improving reliability and reducing cross-browser issues.
The continuous development and strong community support ensure that Selenium will remain a critical tool in the test automation landscape for years.
The bottom line
If you are looking for a powerful and flexible tool for automating your web application testing, then Selenium Testing Tool is the right choice. With Selenium automation testing, you can easily find bugs in your web application and perform efficient and accurate testing on multiple browsers and operating systems. So why wait? Start using Selenium Testing today!
Selenium testing frequently asked questions (FAQs)
Q1: Is Selenium easy to learn?
A: Yes, Selenium is relatively easy to learn compared to other testing tools. There are many online resources available to help(also mentioned in the article).
Q2: What languages can be used with Selenium?
A: You can use many different programming languages with Selenium, including Selenium Java, C#, Python, Selenium Javascript, and Ruby.
Q3: How much does Selenium cost?
A: Selenium is free and open-source software.
Q4: Why is Selenium so widely used?
A: Selenium is used by many companies and organizations because it is a powerful testing tool that is easy to learn and use. The Selenium community is more significant than any other tool in the market, with comprehensive support. This makes the tool highly resourceful and cost-effective for those looking to use it.
Q5: What are the benefits of using Selenium?
A: Some of the key benefits of using Selenium include finding bugs in your web application quickly and easily, automating your web application testing, and running tests on multiple browsers and operating systems simultaneously.
Q6: What’s the difference between Selenium and other testing tools?
A: Selenium is unique from other testing tools because it is open source and can be used with many different programming languages. It’s also very versatile in nature, as it is not just one tool but a set of tools built into a single framework. This framework can be used in any language that supports DOM, giving it an edge over its other counterparts.
Q7: How can we use the Selenium tool with HeadSpin
A: You can use the Selenium tool with HeadSpin to perform automated web and mobile application testing on multiple browsers and operating systems. Using this powerful combination, you can quickly find bugs in your applications, improve the quality of your applications, and optimize your apps for better performance.